『模组开发日记』之暗晶钻套装与工具的加入
本文最后更新于 2024年12月1日 凌晨
暗晶钻装备正式加入世界
引言
因为我是模组开发一半才开始着手开发个人博客,
所有没有从一开始就记录自己的模组开发过程,
不过从现在开始就可以正式记录啦
需要提到一点的是暗晶钻的来源
关于暗晶钻
暗晶钻的合成
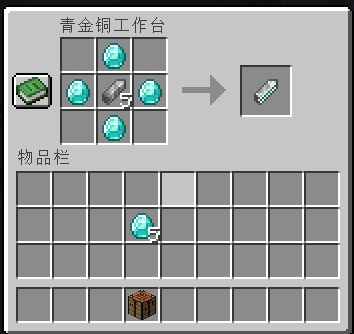
暗晶钻是由一个暗晶碎片加上四个钻石合成的来
关于暗晶碎片
暗晶碎片
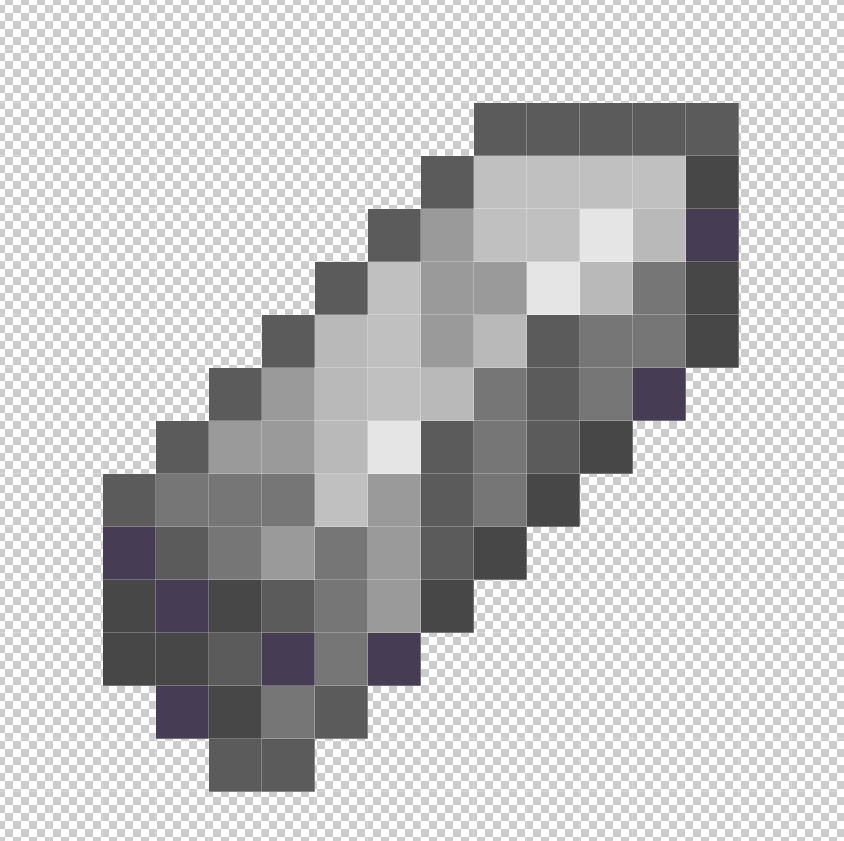
暗晶碎片是开采暗晶簇得来,这是在生存中唯一能得到暗晶碎片的方法
暗晶簇
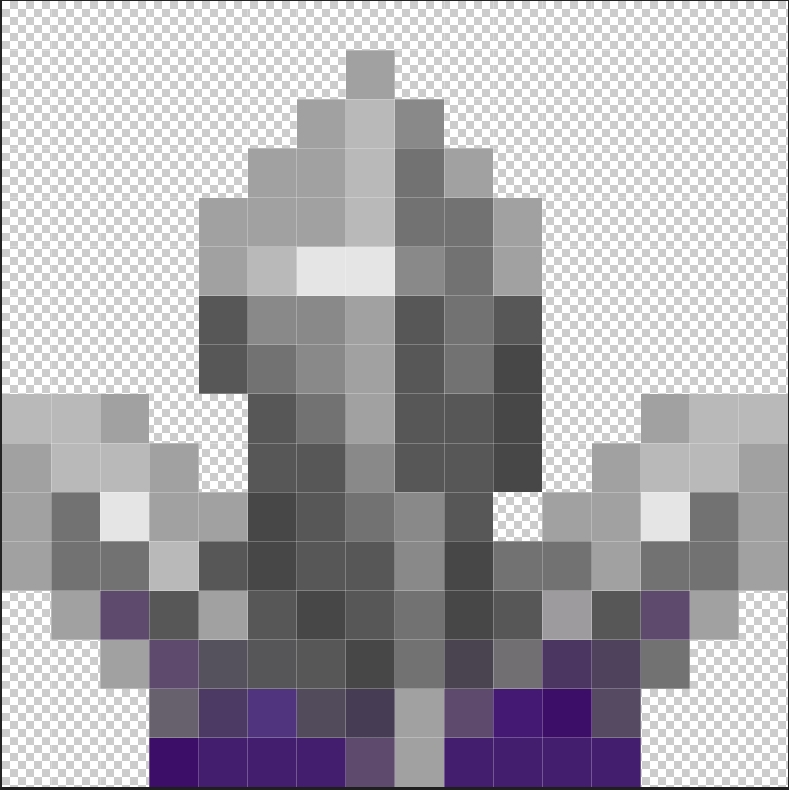
暗晶簇的生成
在主世界的紫水晶洞中,由于环境的不同,紫水晶簇在生长过程中会发生变质而生成一种奇怪又神秘的褪色水晶簇【暗晶簇】
暗晶簇在水晶洞中的状态
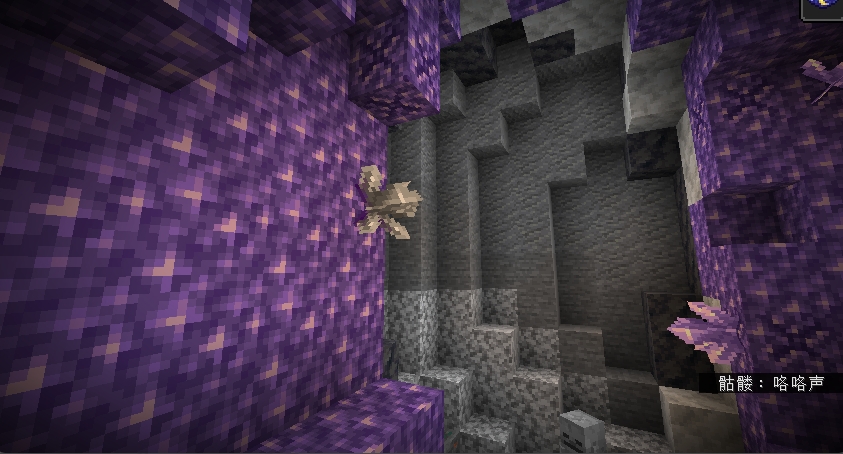
玩家在探索矿洞过程中如果幸运的话可以在紫水晶洞中发现这种神秘的矿石
需要知道的是,这种矿物有且只会在紫水晶洞中生成,且它不会像紫水晶那样会在母岩上再重新长出来
看来是一种很神秘的矿物呢
通过打破暗晶簇可以得到1-3块暗晶碎片
通过上面的合成就可以得到暗晶钻啦
暗晶钻装备
合成
暗晶钻装备的合成和钻石装备合成一模一样
如下所示
暗晶钻头盔的合成
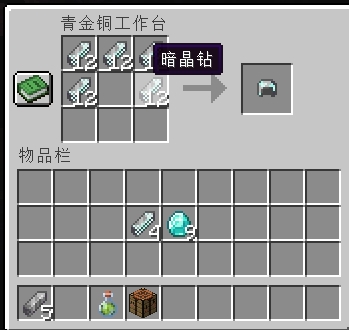
暗晶钻头盔为玩家提供:+3护甲值,+3.5盔甲韧性,+2击退抗性
暗晶钻胸甲的合成
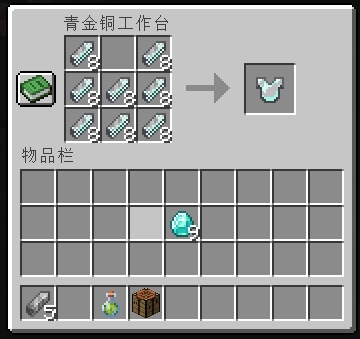
暗晶钻头盔为玩家提供:+8护甲值,+3.5盔甲韧性,+2击退抗性
暗晶钻裤腿的合成
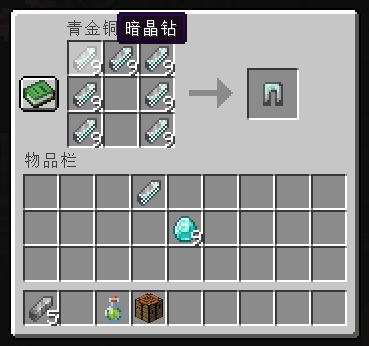
暗晶钻头盔为玩家提供:+6护甲值,+3.5盔甲韧性,+2击退抗性
暗晶钻靴子的合成
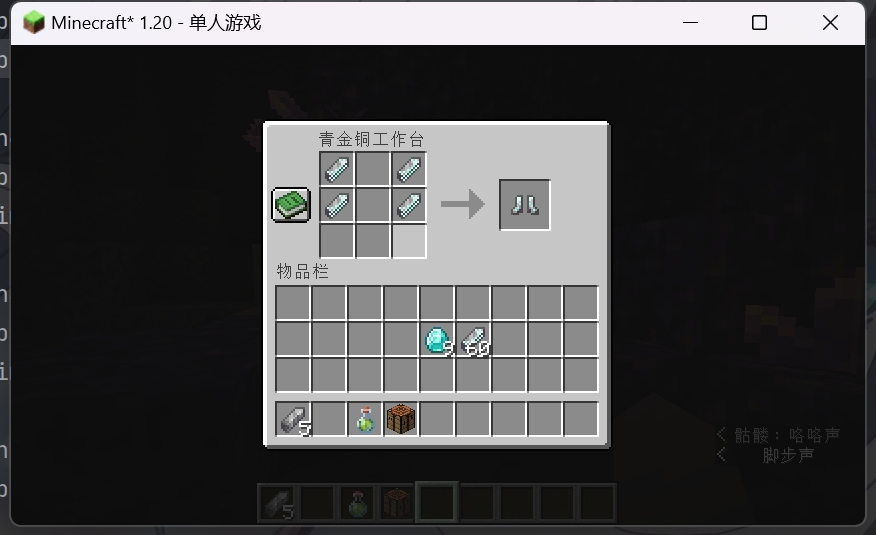
暗晶钻头盔为玩家提供:+3护甲值,+3.5盔甲韧性,+2击退抗性
这样就可以得到暗晶钻四件套了
集齐四件套穿身上会让玩家每15秒获得6颗心的伤害吸收效果
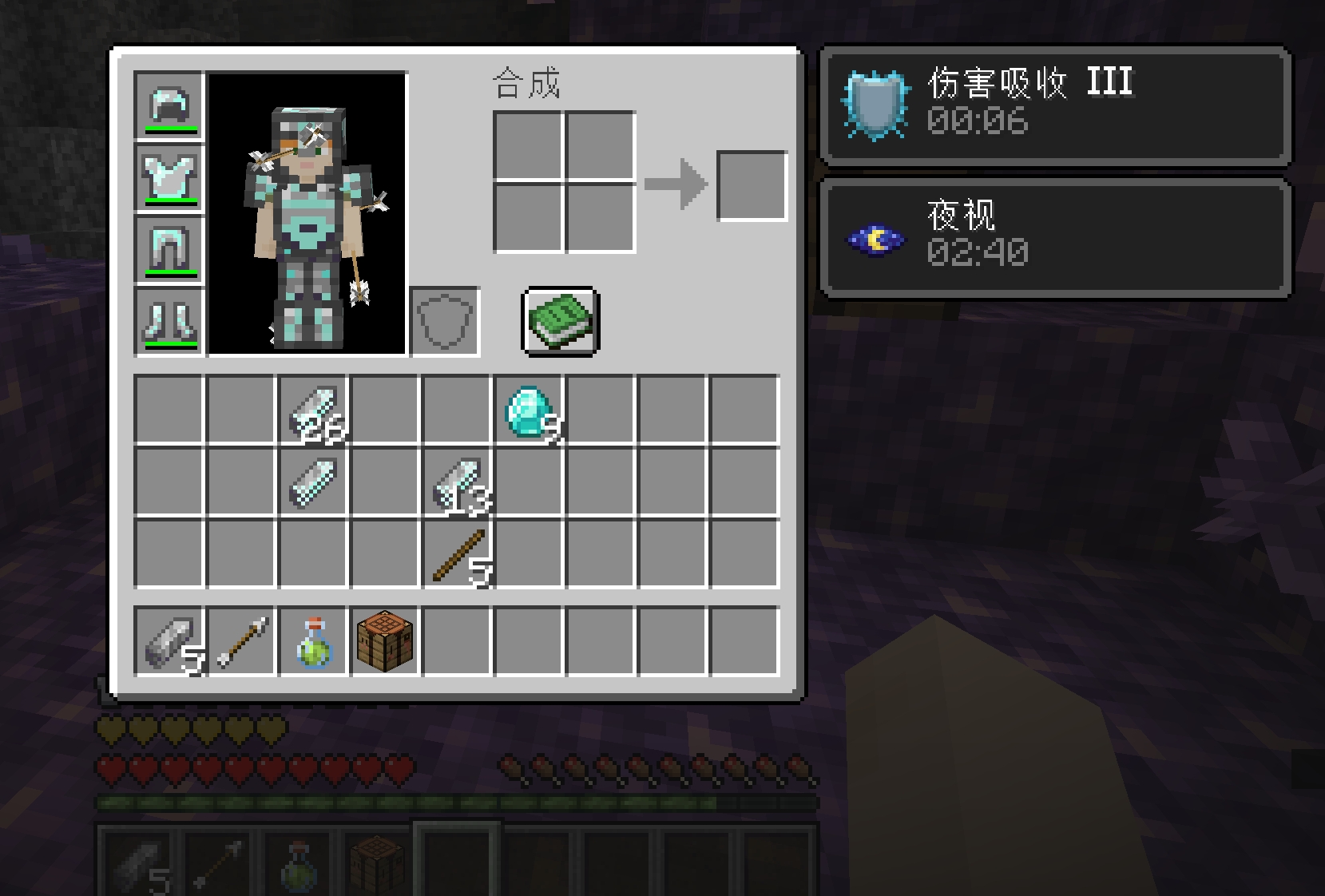
下面是工具类用品的合成
暗晶钻剑的合成
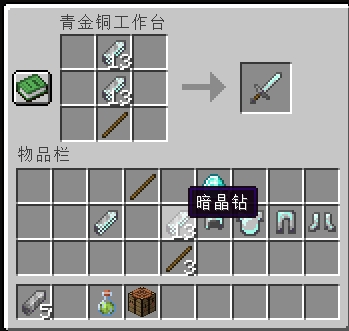
在使用暗晶钻剑攻击生物时会有吸血效果,一般来说每攻击到一个生物会有吸血效果,为自身增加两点生命值,同时攻击多个生物恢复生命值数则按 2*受攻击生物数 来计算
具体代码实现效果如下
1 |
|
暗晶钻斧的合成
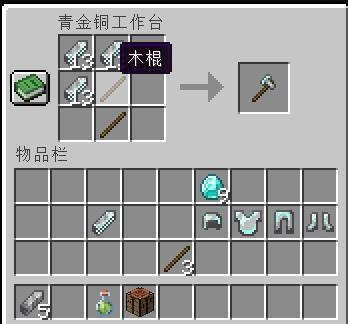
暗晶钻斧头每次使用可以砍伐目标木头上方所有的木头,并且在使用斧头攻击生物时会将生物打出重伤效果(冷却时间五秒钟)
具体代码实现效果如下
1 |
|
暗晶钻镐的合成
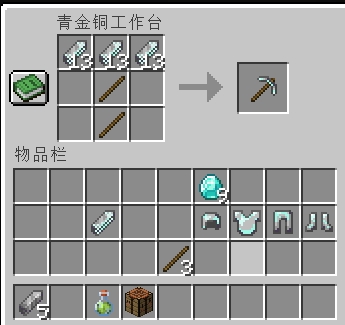
暗晶钻镐使用时右键可以瞬间破坏3*3*3范围内的方块(冷却时间四秒钟)
具体代码实现效果如下
1 |
|
暗晶钻锄的合成
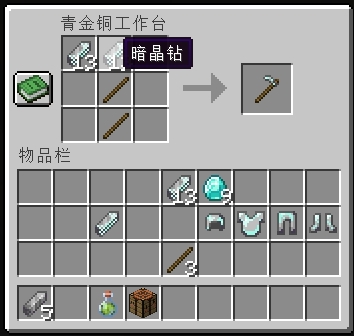
暗晶钻锄使用时可以一次性耕种3*3的农田
具体代码实现效果如下
1 |
|
暗晶钻铲的合成
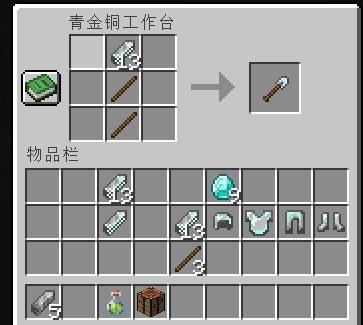
暗晶钻铲暂无特殊功能
以上代码用于 Fabric-1.20 版本模组开发
仅供参考,若想摘抄请在下方留言
总结
该套装开发进程已经完成,后续还会更新
“一花一世界,一叶一菩提”
版权所有 © 2024 云梦泽
欢迎访问我的个人网站:https://hgt12.github.io/